The first thing we learn in Selenium Automation is how to click a button but often we need to click multiple buttons on a webpage. The brute force way to do that is to hardcode it for each button but what if the webpage is dynamic the total number of buttons changes each time? The easiest way to do that is to find all the elements, make a list of them and click them one by one.
There are multiple ways to achieve this task we will go through all of them.
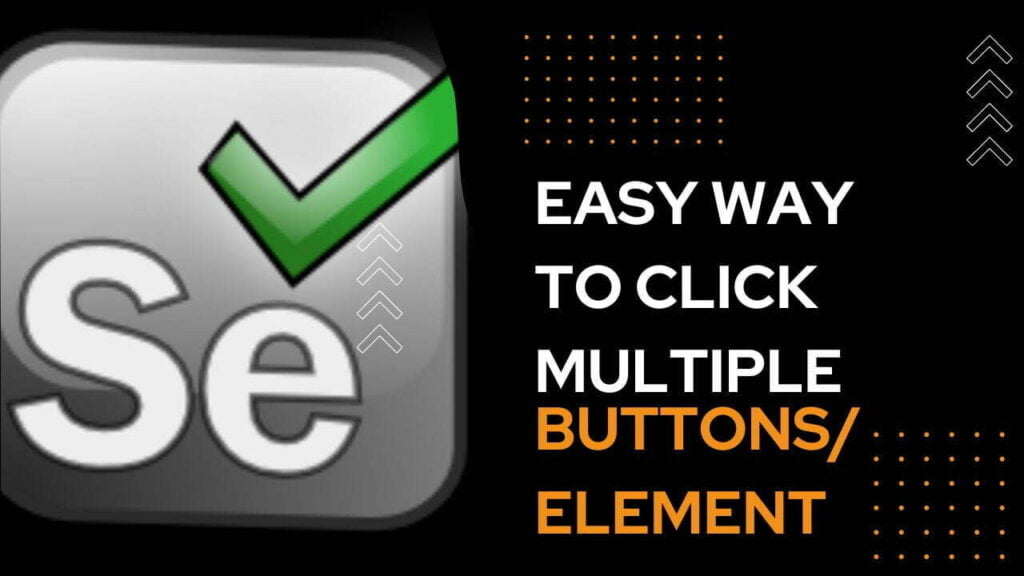
Method 1: Using Class Name
Finding elements using the Class name is one of the easiest ways to locate an element but it was many downsides when many elements belong to the same class or multiple elements have the same class name, in such scenario it fails but if a webpage contains the unique class name or combined unique class name then it’s easier to uniquely identify it.
Don’t forget to import the necessary Header files
from selenium.webdriver.common.by import By
After initializing the necessary header file, we are good to go. Here we will use find_elements() function which is located in the selenium package
elem=driver.find_elements(By.CLASS_NAME, '//Class_Name')
Now “elem” is a list consist all the elements which belong to the same class. In order to click them, we need to itterate through this list and click each element one by one.
for e in elem:
e.click()
Method 2: Using CSS Selector -Selenium
CSS selector is better than class selector since with the help of CSS selector you can easily locate an element.
elem=driver.find_elements(By.CSS_SELECTOR, '//CSS_Selector')
“elem” is a list of elements that has the same selector. In order to click them, we need to iterate over “elem”.
for e in elem
e.click()
Also Read: Create Persistence Kali Linux
Method 3: Using XPATH -Selenium
The path method is the best method with the help of XPath you can easily implement all the other methods and even you can make it more granular.
Initialize Xpath selector,
elem=driver.find_elements(By.XPATH, '//Xpath')
“elem” is a list of elements that have been identified by Xpath. In order to click them, we need to iterate over “elem”.
for e in elem
e.click()
Trick: Learn to easily find Xpath
Final Review
Though there are many methods to identify multiple elements these three methods are the most widely used, out of which “XPath” is best suited since other elements can be easily implemented using Xpath. Personally, I like to use XPath but for faster coding sometimes I use Class Selector and CSS Selector.
Follow me – Pratik Pathak (@pratikpathak.exe) • Instagram photos and videos